Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
Tags
- 스프링 mvc2 - 로그인 처리
- 자바의 정석 기초편 ch8
- 스프링 db1 - 스프링과 문제 해결
- 스프링 mvc1 - 서블릿
- 2024 정보처리기사 시나공 필기
- 자바의 정석 기초편 ch14
- 타임리프 - 기본기능
- 스프링 mvc2 - 타임리프
- 자바의 정석 기초편 ch5
- 스프링 입문(무료)
- 코드로 시작하는 자바 첫걸음
- 자바의 정석 기초편 ch13
- 자바의 정석 기초편 ch3
- 스프링 고급 - 스프링 aop
- 2024 정보처리기사 수제비 실기
- 자바의 정석 기초편 ch1
- 자바의 정석 기초편 ch9
- jpa - 객체지향 쿼리 언어
- 자바의 정석 기초편 ch2
- 자바의 정석 기초편 ch7
- 자바의 정석 기초편 ch11
- 스프링 db2 - 데이터 접근 기술
- 자바의 정석 기초편 ch12
- @Aspect
- 게시글 목록 api
- jpa 활용2 - api 개발 고급
- 스프링 mvc2 - 검증
- 자바의 정석 기초편 ch4
- 자바의 정석 기초편 ch6
- 스프링 mvc1 - 스프링 mvc
Archives
- Today
- Total
나구리의 개발공부기록
자바의 정석 기초편 ch11 - 52 ~ 56[Collections클래스, 컬레션클래스 요약] 본문
유튜브 공부/JAVA의 정석 기초편(유튜브)
자바의 정석 기초편 ch11 - 52 ~ 56[Collections클래스, 컬레션클래스 요약]
소소한나구리 2023. 12. 12. 20:301) Collections클래스
- 컬렉션을 위한 static메서드를 제공, 비슷한 클래스로 Objects(객체 다룰 때) Arrays(배열 다룰 때) 클래스가 있음
(1) 컬렉션 채우기, 복사, 정렬, 검색 메서드
- fill(): 채우기
- copy():복사
- sort(): 정렬
- binarySearch(): 검색
- Arrays클래스의 메서드와 동일하기 때문에 자세한 설명은 제외
(2) 컬렉션의 동기화 - synchronizedXXX()
- synchronized컬렉션명()
- 인수의 콜렉션들을 동기화함
- 필요할때 컬렉션을 동기화하는 메서드임
static Collection synchronizedCollection(Collection c)
static List synchronizedList(List list)
static Set synchronizedSet(Set s)
static Map synchronizedMap(Map m)
static SortedSet synchronizedSortedSet(SortedSet ss)
static SortedMap synchronizedSortedMap(SortedMap sm)
// 동기화가 된 ArrayList(Vector와 기능이 거의 비슷)
List SyncList = Collections.SynchronizedList(New ArrayList(...));
(3) 변경불가(readOnly)컬렉션 만들기 - unmodifiableXXX()
- unmodifiable컬렉션명()
- 인수의 컬렉션을 읽기전용으로 변경
- 컬렉션이 변경되는 것을 방지해야할 때 사용
Static Collection UnmodifiableCollection(Collection c)
Static List UnmodifiableList(List list)
Static Set UnmodifiableSet(Set s)
Static Map UnmodifiableMap(Map m)
Static NavigableSet UnmodifiableNavigableSet(NavigableSet ns)
Static SortedSet UnmodifiableSortedSet(SortedSet ss)
Static NavigableMap UnmodifiableNavigableMap(NavigableMap NM)
Static SortedMap UnmodifiableSortedMap(SortedMap sm)
(4) 싱글톤 컬렉션 만들기 - singletonXXX()
- 반환타입이 Set인 경우에는 singleton(), List와 Map은 singleton컬렉션명()으로 구성되어있음
- 인수의 객체를 반환타입의 싱글톤 객체로 변환하여 반환
- 싱글톤: 객체 1개만 저장가능함
static List singletonList(Object o)
static Set singleton(Object o) // singletonSet이 아님 - 주의!
static Map singletonMap(Object key, Object value)
(5) 한 종류의 객체만 저장하는 컬렉션 만들기 - checked()
- chedcked컬렉션명()
- 1가지 타입만 저장 가능한 컬렉션으로 반환하며, 두번째 인수로 타입을 지정할 수 있음
- 12장에서 배우는 제네릭스(JDK1.5부터 나옴)와 동일하며 현재는 제네릭스를 대부분 사용함
Static Collection checkedCollection(Collection c, Class type)
Static List checkedList(List list, Class type)
Static Set checkedSet(Set s, Class type)
Static Map checkedMap(Map m, Class type, Class valueType)
Static Queue checkedMap(Map m, Class type)
Static NavigableSet checkedNavigableSet(NavigableSet ns, Class type)
Static SortedSet checkedSortedSet(SortedSet ss, Class type)
Static NavigableMap checkedNavigableMap(NavigableMap NM, Class type, Class valueType)
Static SortedMap checkedSortedMap(SortedMap sm, Class type, Class valueType)
// 사용 방법
List list = ArrayList();
List checkedList = checkedList(list, String.class); // String만 저장가능
checkedList.add("abc"); // OK
checkedList.add(new Integer(3)); // 에러. ClassCastException발생
(6) Collections의 주요 메서드 예제
- 상세한 설명은 코드 주석을 참조
import java.util.*;
import static java.util.Collections.*; //Collections를 생략 가능하게 해줌
class Ex11_19 {
public static void main(String[] args) {
List list = new ArrayList();
System.out.println(list);
// 메서드 설명
addAll(list, 1,2,3,4,5); // 요소 저장
System.out.println(list);
rotate(list, 2); // 요소를 오른쪽으로 2번 이동(회전)
System.out.println(list);
swap(list, 0, 2); // 인덱스 0번과 2번의 위치를 변경
System.out.println(list);
shuffle(list); // 저장된 요소의 위치를 임의로 변경
System.out.println(list);
sort(list, reverseOrder()); // 역순 정렬 reverse(list);와 동일
System.out.println(list);
sort(list); // 정렬
System.out.println(list);
//binarySearch전에는 항상 정렬 먼저 진행
int idx = binarySearch(list, 3); // 3이 저장된 위치(index)를 반환
System.out.println("index of 3 = " + idx);
System.out.println("max="+max(list)); // 최대
System.out.println("min="+min(list)); // 최소
System.out.println("min="+max(list, reverseOrder())); // 최소
fill(list, 9); // list를 9로 채운다.
System.out.println("list="+list);
// list와 같은 크기의 새로운 list를 생성하고 2로 채운다. 단, 결과는 변경불가
List newList = nCopies(list.size(), 2);
System.out.println("newList="+newList);
System.out.println(disjoint(list, newList)); // 공통요소가 없으면 true
copy(list, newList); // newList의 값을 list에 복사
System.out.println("newList="+newList);
System.out.println("list="+list);
replaceAll(list, 2, 1); // list의 값중 2를 1로 변경
System.out.println("list="+list);
Enumeration e = enumeration(list); // iterator와 동일
ArrayList list2 = list(e);
System.out.println("list2="+list2);
}
}
/*
출력
[]
[1, 2, 3, 4, 5]
[4, 5, 1, 2, 3]
[1, 5, 4, 2, 3]
[4, 2, 1, 3, 5]
[5, 4, 3, 2, 1]
[1, 2, 3, 4, 5]
index of 3 = 2
max=5
min=1
min=1
list=[9, 9, 9, 9, 9]
newList=[2, 2, 2, 2, 2]
true
newList=[2, 2, 2, 2, 2]
list=[2, 2, 2, 2, 2]
list=[1, 1, 1, 1, 1]
list2=[1, 1, 1, 1, 1]
*/
2) 컬렉션 클래스 정리 & 요약
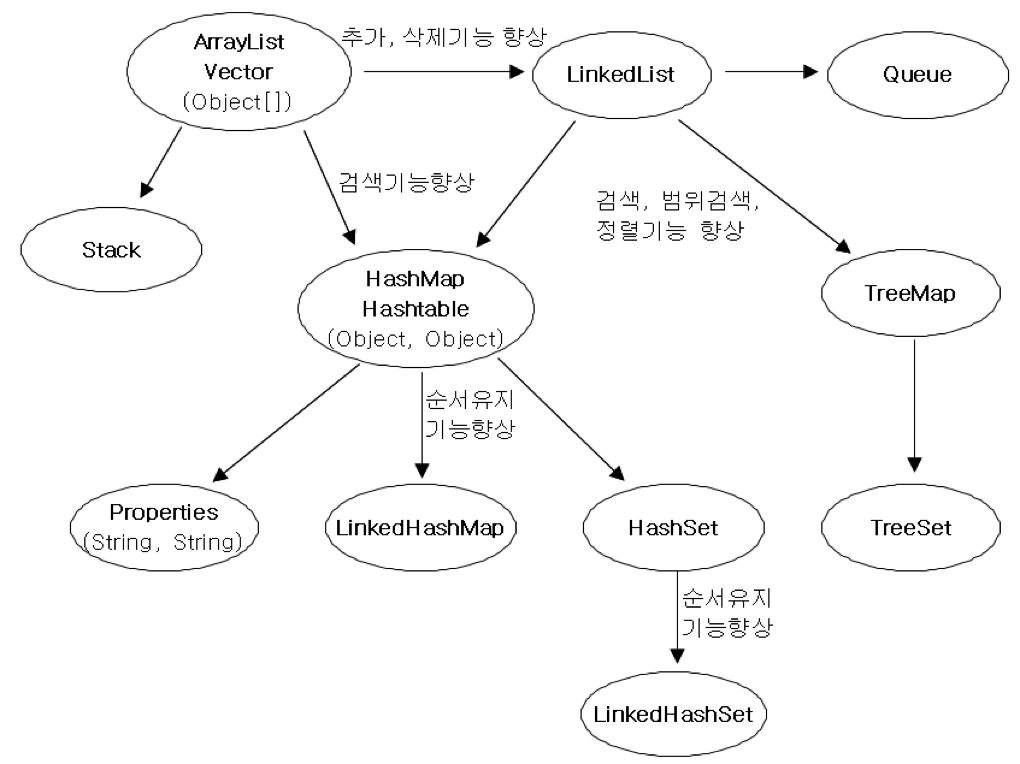
(1) ArrayList
- 배열기반
- 데이터의 추가와 삭제에 불리하지만 순차적인 추가/삭제는 제일빠름
- 임의의 요소에 대한 접근성이 뛰어남
(2) LinkedList
- 연결기반
- 데이터의 추가와 삭제에 유리하지만 임의의 요소에 대한 접근성이 좋지 않음
(3) HashMap
- 배열과 연결이 결합된 형태
- 추가, 삭제, 검색, 접근성이 모두 뛰어나며 검색에는 최고성능을 보임
(4) TreeMap
- 연결기반
- 정렬과 검색(특히 범위검색)에 적합하며 일반적인 검색성능은 HashMap보다 떨어짐
(5) Stack
- Vector를 상속받아 구현함
- Last In First Out 구조
(6) Queue
- LinkedList가 Queue인터페이스를 구현한 대표적인 구현체임
- First In First Out 구조
(7) Properties
- Hashtable을 상속받아 구현됨
- String, String 구조로 되어있음
(8) HashSet
- HashMap을 이용하여 구현됨
(9) TreeSet
- TreeMap을 이용해서 구현됨
(10) LinkedHashMap, LinkedHashSet
- HashMap과 HashSet에 저장순서유지기능을 추가함
** 출처 : 남궁성의 정석코딩_자바의정석_기초편 유튜브 강의